Xây dựng chương trình xem video trên hệ điều hành Android. Việc tạo ra chương trình xem video không có gì là khó, đơn giản chỉ là xử lý file, list, view các button, sdcard, đối tượng media VideoView như bài audio mà hôm trước chúng ta đã cùng nghiên cứu.
Bước 1: Xây dựng giao diện main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:padding="20dip">
<LinearLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:paddingLeft="20dip"
android:paddingRight="20dip">
<TextView
android:text="@string/hello"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginBottom="20dip"
android:textSize="20sp"
android:textStyle="italic" android:gravity="center"/>
<TableLayout
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_gravity="center"
android:stretchColumns="*">
<TableRow>
<ListView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@id/android:list"
android:layout_width="wrap_content"
android:layout_height="100dp"
android:scrollbarAlwaysDrawVerticalTrack="true"
android:scrollbars="vertical" >
</ListView>
</TableRow>
<TableRow >
<VideoView
android:id="@+id/video_frame"
android:layout_width="wrap_content"
android:layout_height="150dp"
android:layout_gravity="center"
android:visibility="visible"/>
</TableRow>
</TableLayout>
<TableLayout
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="50dip"
android:stretchColumns="*">
<TableRow>
<Button
android:id="@+id/play_button"
android:text="@string/play_label" android:visibility="invisible"/>
<Button
android:id="@+id/stop_button"
android:text="@string/stop_label" android:visibility="invisible" />
</TableRow>
<TableRow>
<Button
android:id="@+id/about_button"
android:text="@string/about_label" />
<Button
android:id="@+id/exit_button"
android:text="@string/exit_label" />
</TableRow>
</TableLayout>
</LinearLayout>
</LinearLayout>
Bước 2: Tạo chuỗi strings.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<!-- Basic -->
<string name="hello">Welcome to Video!</string>
<string name="app_name">Video</string>
<!-- Control -->
<string name="play_label">Play</string>
<string name="pause_label">Pause</string>
<string name="stop_label">Stop</string>
<string name="about_label">About</string>
<string name="exit_label">Exit</string>
<!-- About -->
<string name="about_title">About this Application</string>
<string name="about_text">
<b>Create by:</b>\n
Lê Thiện Nhật Quang (11TLT)\n
Võ Hoài Sơn
</string>
<!-- Other -->
</resources>
Bước 3: Tạo lớp Video.java
package quangit.video.videoactive;
import java.io.File;
import java.io.FilenameFilter;
import java.util.ArrayList;
import java.util.List;
import android.app.ListActivity;
import android.content.Context;
import android.content.Intent;
import android.os.Bundle;
import android.util.AttributeSet;
import android.util.Log;
import android.view.KeyEvent;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.ListView;
import android.widget.MediaController;
import android.widget.Toast;
import android.widget.VideoView;
public class Video extends ListActivity implements OnClickListener{
// Interface components
private View playButton, stopButton, aboutButton, exitButton;
// Store list Videos
private List<String> listFilesApp = new ArrayList<String>();
// Position of a Video in list
private int position=-1; // Not choose any Video
// Path of Videos Folder
private static final String pathFilesApp="/sdcard/Video/";
// Media Object
private VideoView mp;
/**-----------------------------------------------------------------
* Application's basic methods
* ----------------------------------------------------------------- */
@Override
public void onCreate(Bundle savedInstanceState) {
// TODO Auto-generated method stub
super.onCreate(savedInstanceState);
// Call layout main.xml
setContentView(R.layout.main);
// Search View
playButton = this.findViewById(R.id.play_button);
stopButton = this.findViewById(R.id.stop_button);
aboutButton = this.findViewById(R.id.about_button);
exitButton = this.findViewById(R.id.exit_button);
// Setup click listeners for all the buttons
playButton.setOnClickListener(this);
stopButton.setOnClickListener(this);
aboutButton.setOnClickListener(this);
exitButton.setOnClickListener(this);
// Fill view from resource
mp = (VideoView)findViewById(R.id.video_frame);
// Add list Videos to layout
addVideosToList();
ArrayAdapter<String> t_l = new ArrayAdapter<String>(this, R.layout.item, listFilesApp);
setListAdapter(t_l);
//mp.setVideoPath("/sdcard/Movies/Grapes to Raisins Time Lapse.3gp" );
//mp.start();
}
@Override
protected void onStart() {
// TODO Auto-generated method stub
super.onStart();
}
@Override
protected void onRestart() {
// TODO Auto-generated method stub
super.onRestart();
}
@Override
protected void onPause() {
// TODO Auto-generated method stub
super.onPause();
}
@Override
protected void onResume() {
// TODO Auto-generated method stub
super.onResume();
}
@Override
protected void onStop() {
// TODO Auto-generated method stub
super.onStop();
}
@Override
protected void onDestroy() {
// TODO Auto-generated method stub
super.onDestroy();
}
public void exitApp(){
stopVideo();
Intent i_exit = new Intent(Intent.ACTION_MAIN);
i_exit.addCategory(Intent.CATEGORY_HOME);
i_exit.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
startActivity(i_exit);
}
/**-------------------------------------------------------------
* Events handler methods
* ------------------------------------------------------------- */
// Handling buttons events
public void onClick(View v) {
switch (v.getId()) {
case R.id.play_button:
pauseVideo();
break;
case R.id.stop_button:
stopVideo();
break;
case R.id.about_button:
Intent i_about = new Intent(this, VideoActivity.class);
startActivity(i_about); //Set activity for class About.java
break;
case R.id.exit_button:
exitApp();
break;
default:
break;
}
}
// Handling keyboard events
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
// TODO Auto-generated method stub
switch (keyCode) {
case KeyEvent.KEYCODE_BACK:
exitApp();
break;
case KeyEvent.KEYCODE_HOME:
exitApp();
break;
default:
return super.onKeyDown(keyCode, event);
}
return true;
/**
if (keyCode == event.KEYCODE_BACK){
finish(); // Exit Video
return true;
}
return super.onKeyDown(keyCode, event);
*/
}
/**-----------------------------------------------------
* Video application methods
* ----------------------------------------------------- */
// Class File Filter
class FileFilter implements FilenameFilter{
@Override
public boolean accept(File dir, String filename) {
// TODO Auto-generated method stub
return (filename.endsWith(".3gp"));
}
}
// Add Videos to list
public void addVideosToList(){
File t_f = new File(pathFilesApp);
for (File file: t_f.listFiles(new FileFilter())) {
listFilesApp.add(file.getName());
}
}
// Click a Video on list Videos
@Override
protected void onListItemClick(ListView _l, View _v, int _p, long _id) {
// TODO Auto-generated method stub
super.onListItemClick(_l, _v, _p, _id);
// Play the Video is clicked ~ It is the current Video is playing
playVideo(pathFilesApp + listFilesApp.get(_p));
// Save position of current Video is playing
position = _p;
// Show info of the current Video is playing
Toast.makeText(getApplicationContext(), listFilesApp.get(_p), Toast.LENGTH_LONG).show();
}
// Play a Video
public void playVideo(String _path){
try {
mp.setVideoPath(_path);
//mp.setMediaController(new MediaController(this));
MediaController mc = new MyMediaController(mp.getContext());
mc.setMediaPlayer(mp);
mp.setMediaController(mc);
mp.requestFocus();
mp.start();
// Label button
Button t_bt = (Button)playButton;
t_bt.setText("Pause");
// Auto play next Video
}catch(Exception e){
// TODO: handle exception
Log.v("VideoPlayer", e.getMessage());
}
}
// Pause Video
public void pauseVideo(){
if(mp.isPlaying()){
mp.pause();
Button t_bt = (Button)playButton;
t_bt.setText("Play");
}else{
if(position != -1){
mp.start();
Button t_bt = (Button)playButton;
t_bt.setText("Pause");
}
}
}
// Stop Video
public void stopVideo(){
if(mp.isPlaying()){
mp.stopPlayback();
position=-1; // Not choose any Video
Button t_bt = (Button)playButton;
t_bt.setText("Play");
}
}
// Restart Video
public void restartVideo(){
mp.seekTo(0); // Reset Video's time line
}
// Play next Video
public void playNextVideo(){
if(++position >= listFilesApp.size()){
position=0;
}else{
playVideo(pathFilesApp + listFilesApp.get(position)) ;
}
}
}
class MyMediaController extends MediaController {
public MyMediaController(Context context, AttributeSet attrs) {
super(context, attrs);
}
public MyMediaController(Context context, boolean useFastForward) {
super(context, useFastForward);
}
public MyMediaController(Context context) {
super(context);
}
@Override
public void show(int timeout) {
super.show(0);
}
}
Bước 4: Import file video vào trong sdcard như trong bài Audio. Sau đó Run Application để xem thành quả
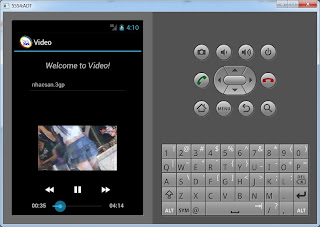