Thiết kế class Hóa Đơn đáp ứng yêu cầu xử lý của giao diện
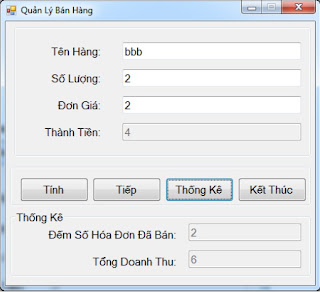
Lớp HoaDon
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Bai01
{
class HoaDon
{
private String tenhang;
private double soluong;
private double dongia;
public static double sohoadon = 0;
public static double doanhthu = 0;
public string Tenhang
{
get { return tenhang; }
set { tenhang = value; }
}
public double Soluong
{
get { return soluong; }
set { soluong = value; }
}
public double Dongia
{
get { return dongia; }
set { dongia = value; }
}
public static double Sohoadon
{
get { return sohoadon; }
}
public static double Doanhthu
{
get { return doanhthu; }
}
public HoaDon()
{
sohoadon = 0;
doanhthu = 0;
}
public HoaDon(String tenhang,double soluong,double dongia)
{
this.tenhang = tenhang;
this.soluong = soluong;
this.dongia = dongia;
sohoadon++;
}
public double thanhTien()
{
double thanhTien = this.soluong*this.dongia;
doanhthu += thanhTien;
return thanhTien;
}
public double getSoHoaDon()
{
return sohoadon;
}
public double getDoanhThu()
{
return doanhthu;
}
}
}
Lớp Form1
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Bai01
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private double soLuong, donGia;
private HoaDon h;
bool kiemTraTenHang()
{
if (string.IsNullOrEmpty(txtTenHang.Text))
{
MessageBox.Show("chưa nhập tên hàng");
txtTenHang.Focus();
return false;
}
return true;
}
bool kiemTraSoLuong()
{
try
{
if (string.IsNullOrEmpty(txtSoLuong.Text))
{
MessageBox.Show("chưa nhập số lượng");
txtSoLuong.Focus();
return false;
}
else
{
soLuong = double.Parse(txtSoLuong.Text);
if (soLuong <= 0) throw new Exception();
}
}
catch (Exception)
{
MessageBox.Show("Số Lượng Không Hợp Lệ");
txtSoLuong.SelectAll();
txtSoLuong.Focus();
return false;
}
return true;
}
bool kiemTraDonGia()
{
try
{
if (string.IsNullOrEmpty(txtDonGia.Text))
{
MessageBox.Show("chưa nhập Đơn Giá");
txtDonGia.Focus();
return false;
}
else
{
donGia = double.Parse(txtDonGia.Text);
if (donGia <= 0) throw new Exception();
}
}
catch (Exception)
{
MessageBox.Show("Đơn Giá Không Hợp Lệ");
txtDonGia.SelectAll();
txtDonGia.Focus();
return false;
}
return true;
}
private void btnTinh_Click(object sender, EventArgs e)
{
if(kiemTraTenHang())
{
if(kiemTraSoLuong())
{
if(kiemTraDonGia())
{
h = new HoaDon(txtTenHang.Text, soLuong, donGia);
txtThanhTien.Text = h.thanhTien().ToString();
}
}
}
}
private void btnTiep_Click(object sender, EventArgs e)
{
txtTenHang.Clear();
txtSoLuong.Clear();
txtDonGia.Clear();
txtThanhTien.Clear();
txtTenHang.Focus();
}
private void btnThongKe_Click(object sender, EventArgs e)
{
txtSoHoaDon.Text = h.getSoHoaDon().ToString();
txtTongDoanhThu.Text = h.getDoanhThu().ToString();
}
private void btnKetThuc_Click(object sender, EventArgs e)
{
this.Close();
}
}
}